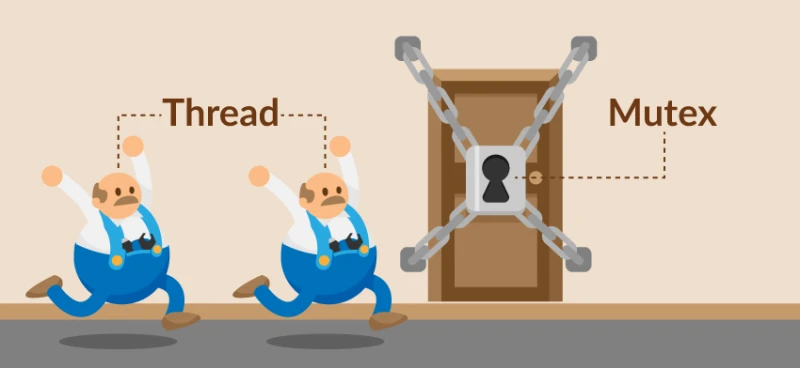
Mutex و Semaphore در Thread
Mutex چیست؟
Mutex مشابه یک قفل در CSharp برای همگام سازی کار می کند اما بین چندین پردازش کار میکند. Mutex در مقابل thread های خارجی حالت امن ایجاد می کند.
Semaphore چیست؟
Semaphore به یک یا چند thread اجازه می دهد تا به صورت thread safe وارد task خود شده و آن را اجرا کنند. شی کلاس Semaphore دو پارامتر می گیرد. اولی تعداد فرآیندها برای شروع اولیه و دومی برای تعریف حداکثر تعداد فرآیندهایی است که می توانند برای شروع اولیه استفاده شوند. پارامتر دوم باید مساوی یا بزرگتر از پارامتر اول باشد.
مثال Mutex
using System;
using System.Threading;
namespace threading
{
class Mutex_Example
{
private static Mutex mutex = new Mutex();
public void Example()
{
//Create mumber of thread to explain muiltiple thread example
for (int i = 0; i < 4; i++)
{
Thread t = new Thread(MutexDemo);
t.Name = string.Format("Thread {0} :", i + 1);
t.Start();
}
}
static void Main(string[] args)
{
Mutex_Example p = new Mutex_Example();
p.Example();
Console.ReadKey();
}
//Method to implement syncronization using Mutex
static void MutexDemo()
{
try
{
//Blocks the current thread until the current WaitHandle receives a signal.
mutex.WaitOne(); // Wait until it is safe to enter.
Console.WriteLine("{0} has entered in the Domain", Thread.CurrentThread.Name);
Thread.Sleep(1000); // Wait until it is safe to enter.
Console.WriteLine("{0} is leaving the Domain\r\n", Thread.CurrentThread.Name);
}
finally
{
//ReleaseMutex unblock other threads that are trying to gain ownership of the mutex.
mutex.ReleaseMutex();
}
}
}
}
مثال Semaphore
using System;
using System.Threading;
namespace threading
{
class Semaphore_Example
{
//Object of Semaphore class with two parameter.
//first parameter explain number of process to initial start
//second parameter explain maximum number of process can start
//second parameter must be equal or greate then first paramete
static Semaphore obj = new Semaphore(3, 2);
static void Main(string[] args)
{
for (int i = 1; i <= 5; i++)
{
Thread t = new Thread(SempStart);
t.Start(i);
}
Console.ReadKey();
}
static void SempStart(object id)
{
Console.WriteLine(id + " Wants to Get Enter for processing");
try
{
//Blocks the current thread until the current WaitHandle receives a signal.
obj.WaitOne();
Console.WriteLine(" Success: " + id + " is in!");
Thread.Sleep(5000);
Console.WriteLine(id + "<<-- is exit because it completed there operation");
}
finally
{
//Release() method to releage semaphore
obj.Release();
}
}
}
}
منبع:
https://www.c-sharpcorner.com/blogs/mutex-and-semaphore-in-thread